- Namespaces
- Nodes
- Workloads
- Deployments
- StatefulSets
- DaemonSets
- Jobs
- CronJobs
- Pods
- Network
- Services
- Endpoints
- Ingress
- Storage
- Persistent Volumes
- Persistent Volume Claims
- Storage Classes
- Configuration
- Config Maps
- Secrets
- Custom resources
- Helm
K8s Architecture and Components
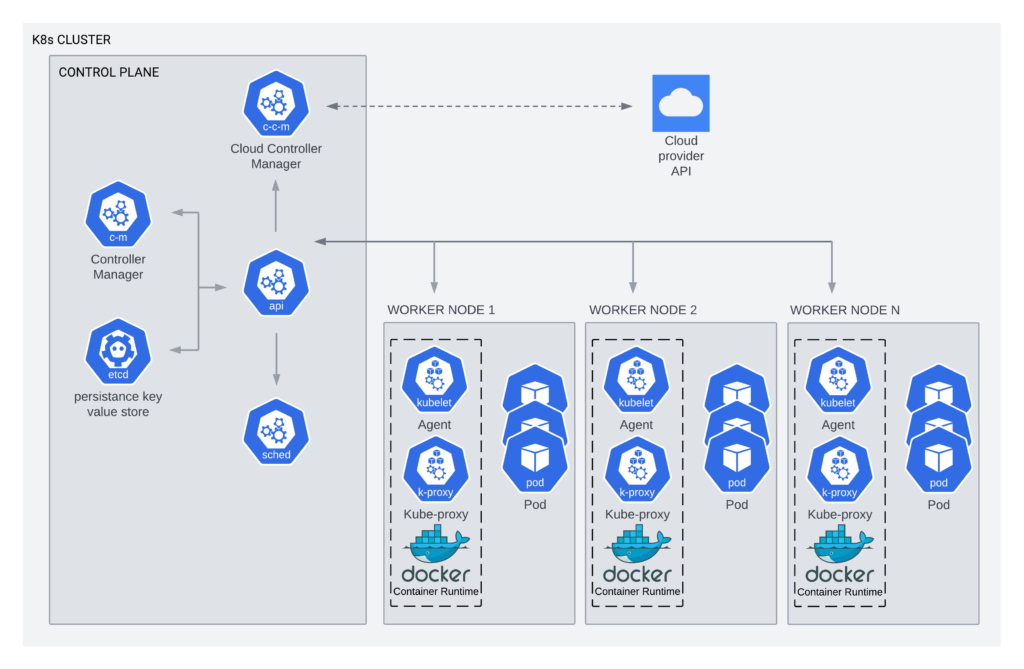
Controllers
Replication controller
ensures that a specified number of replicas of a pod are running at all times
Deployment controller
extends the functionality of Replication Controller and provides features such as rolling updates and rollbacks
StatefulSet controller
used to manage stateful applications and maintains the uniqueness of each pod
DaemonSet controller
used to ensure that all or some of the nodes run a copy of a specific pod
Job and CronJob controllers
used to run one-time or recurring batch jobs in a cluster
Service controller
used to expose a set of pods to the network as a single endpoint
Horizontal Pod Autoscaler
automatically scales the number of pods in a deployment based on resource usage
Ingress controller
used to handle external traffic to the services in a cluster
K8s manifest files examples:
Pod
Pod represents a single instance of a running process in a cluster
apiVersion: v1
kind: Pod
metadata:
name: nginx-pod
spec:
containers:
- name: nginx
image: nginx:latest
ReplicaSet
ReplicaSet is low-level abstraction & ensures that a specified # of replicas of a Pod are running at any given time
apiVersion: apps/v1
kind: ReplicaSet
metadata:
name: nginx-replica-set
spec:
replicas: 10
selector:
matchLabels:
app: nginx
template:
metadata:
labels:
app: nginx
spec:
containers:
- name: nginx
image: nginx:latest
Deployment
Deployment is high-level abstraction that manages ReplicaSets and provides additional features such as rolling updates and rollbacks
apiVersion: apps/v1
kind: Deployment
metadata:
name: nginx-deployment
spec:
replicas: 10
selector:
matchLabels:
app: nginx
template:
metadata:
labels:
app: nginx
spec:
containers:
- name: nginx
image: nginx:latest
Service
Service provides a stable endpoint for a set of Pods.
apiVersion: v1
kind: Service
metadata:
name: nginx-service
spec:
selector:
app: nginx
ports:
- name: http
protocol: TCP
port: 80
targetPort: 80
type: ClusterIP
Service types:
1. ClusterIP (default)
2. NodePort
3. LoadBalancer
4. ExternalName
5. ExternalIP
6. Headless
Ingress
Ingress provides external access to the Services in a cluster
apiVersion: networking.k8s.io/v1
kind: Ingress
metadata:
name: nginx-ingress
spec:
rules:
- host: example.com
http:
paths:
- path: /nginx
pathType: Prefix
backend:
service:
name: nginx-service
port:
name: http
ConfigMap
ConfigMap stores configuration data for Pods and other resources in a cluster
apiVersion: v1
kind: ConfigMap
metadata:
name: nginx-config
data:
nginx.conf: |
server {
listen 80;
}
Secret
Secret stores sensitive data such as passwords and tokens for Pods and other resources in a cluster
apiVersion: v1
kind: Secret
metadata:
name: nginx-secret
type: Opaque
data:
password: YWRtaW4=
token: Zm9vYmFy
StatefulSet
StatefulSet is used to manage stateful applications and ensures that each Pod has a unique identity and stable network identity
apiVersion: apps/v1
kind: StatefulSet
metadata:
name: web-stateful-set
spec:
serviceName: "nginx"
replicas: 2
selector:
matchLabels:
app: nginx
template:
metadata:
labels:
app: nginx
spec:
containers:
- name: nginx
image: nginx:latest
DaemonSet
DaemonSet ensures that a copy of a Pod is running on all or a specified subset of nodes in a cluster
apiVersion: apps/v1
kind: DaemonSet
metadata:
name: fluentd-daemon-set
spec:
selector:
matchLabels:
app: fluentd
template:
metadata:
labels:
app: fluentd
spec:
containers:
- name: fluentd
image: fluentd:latest
Job
Job creates one or more Pods and ensures that a specified number of them successfully complete
apiVersion: batch/v1
kind: Job
metadata:
name: my-job
spec:
template:
spec:
containers:
- name: my-container
image: my-image
restartPolicy: Never
backoffLimit: 4
CronJob
A CronJob creates Jobs on a time-based schedule
apiVersion: batch/v1beta1
kind: CronJob
metadata:
name: my-cron-job
spec:
schedule: "*/1 * * * *"
jobTemplate:
spec:
template:
spec:
containers:
- name: my-container
image: my-image
restartPolicy: OnFailure
ServiceAccount
ServiceAccount provides an identity for processes that run in a Pod
apiVersion: v1
kind: ServiceAccount
metadata:
name: my-service-account
Role
Role defines a set of permissions that can be granted to a ServiceAccount
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
name: my-role
rules:
- apiGroups: ["", "extensions", "apps"]
resources: ["pods", "services"]
verbs: ["get", "list", "watch"]
RoleBinding
RoleBinding grants a Role to a ServiceAccount or group of users
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: my-role-binding
subjects:
- kind: ServiceAccount
name: my-service-account
namespace: default
roleRef:
kind: Role
name: my-role
apiGroup: rbac.authorization.k8s.io
- Namespace: a virtual cluster within a cluster
- PersistentVolume: a piece of storage in the cluster
- PersistentVolumeClaim: a request for storage by a user
- HorizontalPodAutoscaler: automatically scale the number of pods in a deployment based on CPU usage.