PEP – Python Enhancement Proposals
https://www.python.org/dev/peps
- create myscript.py and make it executable and include:
#!/usr/bin/env python3
List, Tuple, Set
- List
[ ] or list()
turtles = ['michelangelo', 'raphael', 'leonardo', 'donatello']
turtles.append("mika") # add record
turtles.pop(1) # remove 2nd record
- Tuple
( ) or tuple()
foods = ("pizza", "shakshuka", "raclette", "salad")
print(len(foods))
- Set
{ } or set()
- Dictionary
{ } or dict() with key pairs
turtles = {'michelangelo':'party dud', 'raphael':'cool but rude', 'leonardo':'leader', 'donatello':'geek'}
print(turtles['leonardo']) # print specific value
del turtles['michelangelo'] # delete record
turtles["shredder"] = "mean dude" # add record
print(turtles)
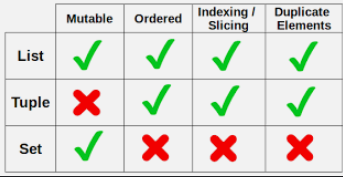
Misc.
type() # get object type
- get help
help()
help(print)
help(list)
help(int())
- string
msg = "this is a string"
print(msg[3]) # will print the 4th character
print(msg[1:5]) # will print the range
print(msg.upper()) # to uppercase
if, elif
age = input("enter your age: \n")
if int(age) >= 50:
print("i am old!")
elif int(age) >= 18:
print("in between!")
else:
print("I am so young!")
while
my_num = 1
while my_num <= 10:
print(my_num)
my_num = my_num + 1
for
foods = ["pizza", "shakshuka", "raclette", "salad"]
for f in foods:
print(f)